This is a blog about embedding a simple java applet inside a web page. A java applet is actually a runnable java program that can be embedded in a website for showing some graphics or making the web page interactive. It all runs by itself and any modern web browser with java plugin can run applets. So we create a sample java program first and then compile it to make the .class file ie the runnable. Then this runnable is embedded in the web page in a mechanism similar to embedding pictures inside the html file using html tags.
So before you starting the applet programming you need a java programming environment within your system including javac compiler and Java Runtime Environment.
I started doing the applet programming as it is a topic in my Web Commerce paper. Still i didn't got enough time to invest for it, but here is the basic things that I came across while discovering the kick of applets with my ubuntu box...
1. First open up your text editor and paint all the code inside it, save it with .java extension. Use the code snippet below or download from here,
import java.applet.*;
import java.awt.*;
public class DrawingStuff extends Applet {
int width, height;
public void init() {
width = getSize().width;
height = getSize().height;
setBackground( Color.black );
}
public void paint( Graphics g ) {
g.setColor( Color.red );
g.drawRect( 10, 20, 100, 15 );
g.setColor( Color.pink );
g.fillRect( 240, 160, 40, 110 );
g.setColor( Color.blue );
g.drawOval( 50, 225, 100, 50 );
g.setColor( Color.orange );
g.fillOval( 225, 37, 50, 25 );
g.setColor( Color.yellow );
g.drawArc( 10, 110, 80, 80, 90, 180 );
g.setColor( Color.cyan );
g.fillArc( 140, 40, 120, 120, 90, 45 );
g.setColor( Color.magenta );
g.fillArc( 150, 150, 100, 100, 90, 90 );
g.setColor( Color.black );
g.fillArc( 160, 160, 80, 80, 90, 90 );
g.setColor( Color.green );
g.drawString( "Groovy!", 50, 150 );
}
}
Code rethink:
To create an applet, you must import the Applet class. This class is in the java.applet package. The Applet class contains code that works with a browser to create a display window
import is a keyword
>java.applet is the name of the package.
>A dot ( . ) separates the package from the class.
>Applet is the name of the class.
>The asterisk, or star (*), means “all classes” in the package.
“awt” stands for “Abstract Window Toolkit”
>The java.awt package includes classes for:
>Drawing lines and shapes
>Drawing letters
>Setting colors
>Choosing fonts
>If it’s drawn on the screen, then java.awt is probably involved!
Your applet class
public class Drawing extends Applet {
.…}
>Drawing is the name of your class
>extends Applet says that our Drawing is a kind of Applet, but with
added capabilities
>Java’s Applet just makes an empty window for drawing
import is a keyword
>java.applet is the name of the package.
>A dot ( . ) separates the package from the class.
>Applet is the name of the class.
>The asterisk, or star (*), means “all classes” in the package.
“awt” stands for “Abstract Window Toolkit”
>The java.awt package includes classes for:
>Drawing lines and shapes
>Drawing letters
>Setting colors
>Choosing fonts
>If it’s drawn on the screen, then java.awt is probably involved!
Your applet class
public class Drawing extends Applet {
.…}
>Drawing is the name of your class
>extends Applet says that our Drawing is a kind of Applet, but with
added capabilities
>Java’s Applet just makes an empty window for drawing
The paint method
>Our applet is needs this method to paint some colored rectangles on
the screen paint needs to be told where on the screen it can draw
>A Graphics (short for “Graphics context”) is an object that holds
information about a painting
>It remembers what color you are using
>It remembers what font you are using
Colors
>The java.awt package defines a class named Color
>There are 13 predefined colors—here are their fully-qualified names:
Color.BLACK Color.PINK Color.GREEN
Color.RED Color.CYANColor.BLUE
Setting a color
>To use a color, we tell our Graphics g what color we want:
>g.setColor(Color.RED);
Java’s coordinate system
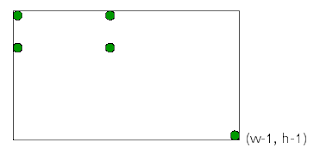
system
>(0, 0) is the top left corner
>(w - 1, h - 1) is just inside the
bottom right corner, where w is
the width of the window and h is
heightDrawing rectangles >There are two ways to draw rectangles:
>g.drawRect( left , top , width , height );
>g.fillRect(left , top , width , height );
Some more java.awt methods >g.drawLine( x1 , y1 , x2 , y2 );
>g.drawOval( left , top , width , height );
>g.fillOval( left , top , width , height );
>g.drawRoundRect( left , top , width , height );
>g.fillRoundRect( left , top , width , height );
>g.drawArc( left , top , width , height ,startAngle , arcAngle );
>g.drawString( string , x , y );
2. Compile the java program to generate the .class file. You can run the folllowing command to compile the java program. You can download the compiled .class file from here if you are still missing a javac compiler
$ javac DrawingStuff.java
3. Create a html file to embed the applet. Save the file with .html extension.
<html>
<body>
<h1>DrawingApplet Applet</h1>
<applet code="DrawingStuff.class"
width="250" height="200">
</applet>
</body>
</html>
4. Copy the .class file to the same folder containing the html file.
That's all .... your sample applet is ready to run. You can see the applet running in your web browser by opening the html file...
0 comments:
Post a Comment
speak out... itz your time !!!